You can download the source code Zip by clicking on the Download Zip button or by visiting GitHub.
You may also like microservices project with spring boot and spring cloud step by step for beginners.
Table of Content:
Introduction
Project Structure
Code
Output
Source Code Location
Video Tutorial Link
Introduction
A project well structured for beginners with deep knowledge on Spring boot, Java, HTML and CSS. we will build a Login and registration page with Backend as Java code and front end using JSP. It is recommended to follow the video tutorial on YouTube and follow step by step development. Project is better viewed on desktop.
Assuming that you have already installed STS and basic development environment setup for a spring project, lets start with creating the project. In STS, Click on Spring started project, Give the project a name and and group ids and include the dependencies “spring-boot-starter-web”, devtools, jpa, “tomcat-embed-jasper”, you can get the dependencies from the pom.xml file below. Ignore the below image for now, it is just to let you know the structure of the files and folders, we will create these files one by one.
Project Structure
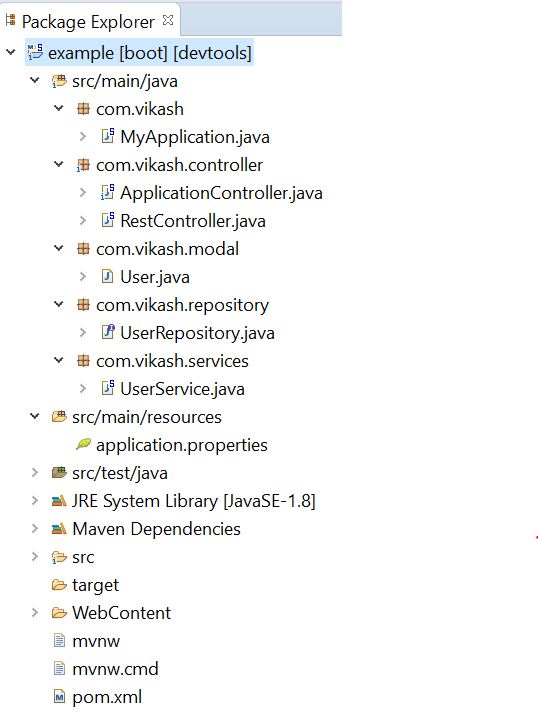
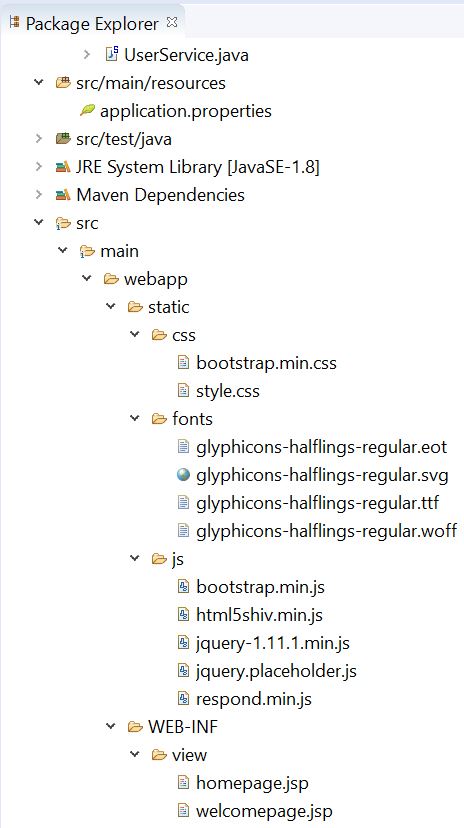
Code
pom.xml
we will start with the pom.xml file which is the entry file for a maven project
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.vikash</groupId> <artifactId>example</artifactId> <version>0.0.1-SNAPSHOT</version> <packaging>jar</packaging> <name>MyApplication</name> <description>Login using Spring MVC</description> <parent> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-parent</artifactId> <version>1.5.8.RELEASE</version> <relativePath /> <!-- lookup parent from repository --> </parent> <properties> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding> <java.version>1.8</java.version> </properties> <dependencies> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-web</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/org.apache.tomcat.embed/tomcat-embed-jasper --> <dependency> <groupId>org.apache.tomcat.embed</groupId> <artifactId>tomcat-embed-jasper</artifactId> <scope>provided</scope> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-starter-tomcat --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-tomcat</artifactId> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-test</artifactId> <scope>test</scope> </dependency> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-data-jpa</artifactId> </dependency> <dependency> <groupId>mysql</groupId> <artifactId>mysql-connector-java</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/javax.servlet/jstl --> <dependency> <groupId>javax.servlet</groupId> <artifactId>jstl</artifactId> </dependency> <!-- https://mvnrepository.com/artifact/javax.servlet/servlet-api --> <dependency> <groupId>javax.servlet</groupId> <artifactId>servlet-api</artifactId> <version>2.5</version> <scope>provided</scope> </dependency> <!-- https://mvnrepository.com/artifact/org.springframework.boot/spring-boot-devtools --> <dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-devtools</artifactId> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-maven-plugin</artifactId> </plugin> </plugins> </build> </project>
When you have created a Spring boot starter project, you will get the basic structure setup, along with that you will get a java file with the main method in it as below.
MyApplication.java
package com.vikash; import org.springframework.boot.SpringApplication; import org.springframework.boot.autoconfigure.SpringBootApplication; @SpringBootApplication public class MyApplication { public static void main(String[] args) { SpringApplication.run(MyApplication.class, args); } }
ApplicationController.java
package com.vikash.controller; import javax.servlet.http.HttpServletRequest; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Controller; import org.springframework.validation.BindingResult; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.ModelAttribute; import org.springframework.web.bind.annotation.PostMapping; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestParam; import com.vikash.modal.User; import com.vikash.services.UserService; @Controller public class ApplicationController { @Autowired UserService userService; @RequestMapping("/welcome") public String Welcome(HttpServletRequest request) { request.setAttribute("mode", "MODE_HOME"); return "welcomepage"; } @RequestMapping("/register") public String registration(HttpServletRequest request) { request.setAttribute("mode", "MODE_REGISTER"); return "welcomepage"; } @PostMapping("/save-user") public String registerUser(@ModelAttribute User user, BindingResult bindingResult, HttpServletRequest request) { userService.saveMyUser(user); request.setAttribute("mode", "MODE_HOME"); return "welcomepage"; } @GetMapping("/show-users") public String showAllUsers(HttpServletRequest request) { request.setAttribute("users", userService.showAllUsers()); request.setAttribute("mode", "ALL_USERS"); return "welcomepage"; } @RequestMapping("/delete-user") public String deleteUser(@RequestParam int id, HttpServletRequest request) { userService.deleteMyUser(id); request.setAttribute("users", userService.showAllUsers()); request.setAttribute("mode", "ALL_USERS"); return "welcomepage"; } @RequestMapping("/edit-user") public String editUser(@RequestParam int id,HttpServletRequest request) { request.setAttribute("user", userService.editUser(id)); request.setAttribute("mode", "MODE_UPDATE"); return "welcomepage"; } @RequestMapping("/login") public String login(HttpServletRequest request) { request.setAttribute("mode", "MODE_LOGIN"); return "welcomepage"; } @RequestMapping ("/login-user") public String loginUser(@ModelAttribute User user, HttpServletRequest request) { if(userService.findByUsernameAndPassword(user.getUsername(), user.getPassword())!=null) { return "homepage"; } else { request.setAttribute("error", "Invalid Username or Password"); request.setAttribute("mode", "MODE_LOGIN"); return "welcomepage"; } } }
RestController.java
package com.vikash.controller; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.GetMapping; import org.springframework.web.bind.annotation.RequestParam; import com.vikash.modal.User; import com.vikash.services.UserService; @org.springframework.web.bind.annotation.RestController public class RestController { @Autowired private UserService userService; @GetMapping("/") public String hello() { return "This is Home page"; } @GetMapping("/saveuser") public String saveUser(@RequestParam String username, @RequestParam String firstname, @RequestParam String lastname, @RequestParam int age, @RequestParam String password) { User user = new User(username, firstname, lastname, age, password); userService.saveMyUser(user); return "User Saved"; } }
User.java
package com.vikash.modal; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.Table; @Entity @Table(name="mytable") public class User { @Id private int id; private String username; private String firstname; private String lastname; private int age; private String password; public User() { } public User(String username, String firstname, String lastname, int age, String password) { super(); this.username = username; this.firstname = firstname; this.lastname = lastname; this.age = age; this.password = password; } public int getId() { return id; } public void setId(int id) { this.id = id; } public String getUsername() { return username; } public void setUsername(String username) { this.username = username; } public String getFirstname() { return firstname; } public void setFirstname(String firstname) { this.firstname = firstname; } public String getLastname() { return lastname; } public void setLastname(String lastname) { this.lastname = lastname; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } @Override public String toString() { return "User [id=" + id + ", username=" + username + ", firstname=" + firstname + ", lastname=" + lastname + ", age=" + age + ", password=" + password + "]"; }}
UserRespoitory.java
package com.vikash.repository; import org.springframework.data.repository.CrudRepository; import com.vikash.modal.User; public interface UserRepository extends CrudRepository<User, Integer> { public User findByUsernameAndPassword(String username, String password); }
UserService.java
package com.vikash.services; import java.util.ArrayList; import java.util.List; import javax.transaction.Transactional; import org.springframework.stereotype.Service; import com.vikash.modal.User; import com.vikash.repository.UserRepository; @Service @Transactional public class UserService { private final UserRepository userRepository; public UserService(UserRepository userRepository) { this.userRepository=userRepository; } public void saveMyUser(User user ) { userRepository.save(user); } public List<User> showAllUsers(){ List<User> users = new ArrayList<User>(); for(User user : userRepository.findAll()) { users.add(user); } return users; } public void deleteMyUser(int id) { userRepository.delete(id); } public User editUser(int id) { return userRepository.findOne(id); } public User findByUsernameAndPassword(String username, String password) { return userRepository.findByUsernameAndPassword(username, password); } }
application.properties
spring.mvc.view.prefix=/WEB-INF/view/ spring.mvc.view.suffix=.jsp #Persistence Related Code spring.datasource.url=jdbc:mysql://localhost/tecnotabyoutube?useSSL=false spring.datasource.username=root spring.datasource.password=root spring.datasource.driver=com.mysql.jdbc.Driver spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5Dialect logging.level.org.hibernate.SQL=debug
Frontend Code
The frontend is written in jsp and there should be a proper folder structure to where these files should be kept or it may not work. Refer the folder structure image at the top of this page and make file accordingly.
homepage.jsp
<!DOCTYPE html > <%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <html> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta http-equiv="Pragma" content="no-cache"> <meta http-equiv="Cache-Control" content="no-cache"> <meta http-equiv="Expires" content="sat, 01 Dec 2001 00:00:00 GMT"> <title>tecno-tab | home</title> <link href="static/css/bootstrap.min.css" rel="stylesheet"> <link href="static/css/style.css" rel="stylesheet"> <!--[if lt IE 9]> <script src="https://oss.maxcdn.com/html5shiv/3.7.3/html5shiv.min.js"></script> <script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script> <![endif]--> </head> <body> <div role="navigation"> <div class="navbar navbar-inverse"> <a href="/welcome" class="navbar-brand">Tecno-Tab</a> <div class="navbar-collapse collapse"> <ul class="nav navbar-nav"> <li><a href="/login">Login</a></li> <li><a href="/register">New Registration</a></li> <li><a href="/show-users">All Users</a></li> </ul> </div> </div> </div> <div class="container" id="homediv"> <div class="jumbotron text-center"> <h1>Welcome to Tecno-tab</h1> <h3>Spring Boot Videos</h3> </div> </div> <div class="container text-centered"> <div class="alert alert-success"> <h4>Session 1: Spring Boot Tutorial</h4> </div> <iframe width="400" height="200" src="https://www.youtube.com/embed/m0Xf6Bf6KFU" frameborder="0" allow="autoplay; encrypted-media" allowfullscreen></iframe> <p> This is 1st Session of the tutorial..... </p> <div class="alert alert-success"> <h4>Session 1: Spring Boot Tutorial</h4> </div> <iframe width="400" height="200" src="https://www.youtube.com/embed/m0Xf6Bf6KFU" frameborder="0" allow="autoplay; encrypted-media" allowfullscreen></iframe> <p> This is 1st Session of the tutorial..... </p> <div class="alert alert-success"> <h4>Session 1: Spring Boot Tutorial</h4> </div> <iframe width="400" height="200" src="https://www.youtube.com/embed/m0Xf6Bf6KFU" frameborder="0" allow="autoplay; encrypted-media" allowfullscreen></iframe> <p> This is 1st Session of the tutorial..... </p> <div class="alert alert-success"> <h4>Session 1: Spring Boot Tutorial</h4> </div> <iframe width="400" height="200" src="https://www.youtube.com/embed/m0Xf6Bf6KFU" frameborder="0" allow="autoplay; encrypted-media" allowfullscreen></iframe> <p> This is 1st Session of the tutorial..... </p> </div> <!-- Optional JavaScript --> <!-- jQuery first, then Popper.js, then Bootstrap JS --> <script src="static/js/jquery-1.11.1.min.js"></script> <script src="static/js/bootstrap.min.js"></script> </body> </html>
welcome.jsp
<!DOCTYPE html > <%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c"%> <html> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta http-equiv="Pragma" content="no-cache"> <meta http-equiv="Cache-Control" content="no-cache"> <meta http-equiv="Expires" content="sat, 01 Dec 2001 00:00:00 GMT"> <title>tecno-tab | home</title> <link href="static/css/bootstrap.min.css" rel="stylesheet"> <link href="static/css/style.css" rel="stylesheet"> <!--[if lt IE 9]> <script src="https://oss.maxcdn.com/html5shiv/3.7.3/html5shiv.min.js"></script> <script src="https://oss.maxcdn.com/respond/1.4.2/respond.min.js"></script> <![endif]--> </head> <body> <div role="navigation"> <div class="navbar navbar-inverse"> <a href="/welcome" class="navbar-brand">Tecno-Tab</a> <div class="navbar-collapse collapse"> <ul class="nav navbar-nav"> <li><a href="/login">Login</a></li> <li><a href="/register">New Registration</a></li> <li><a href="/show-users">All Users</a></li> </ul> </div> </div> </div> <c:choose> <c:when test="${mode=='MODE_HOME' }"> <div class="container" id="homediv"> <div class="jumbotron text-center"> <h1>Welcome to Tecno-tab</h1> <h3>Subscribe my channel to support me</h3> </div> </div> </c:when> <c:when test="${mode=='MODE_REGISTER' }"> <div class="container text-center"> <h3>New Registration</h3> <hr> <form class="form-horizontal" method="POST" action="save-user"> <input type="hidden" name="id" value="${user.id }" /> <div class="form-group"> <label class="control-label col-md-3">Username</label> <div class="col-md-7"> <input type="text" class="form-control" name="username" value="${user.username }" /> </div> </div> <div class="form-group"> <label class="control-label col-md-3">First Name</label> <div class="col-md-7"> <input type="text" class="form-control" name="firstname" value="${user.firstname }" /> </div> </div> <div class="form-group"> <label class="control-label col-md-3">Last Name</label> <div class="col-md-7"> <input type="text" class="form-control" name="lastname" value="${user.lastname }" /> </div> </div> <div class="form-group"> <label class="control-label col-md-3">Age </label> <div class="col-md-3"> <input type="text" class="form-control" name="age" value="${user.age }" /> </div> </div> <div class="form-group"> <label class="control-label col-md-3">Password</label> <div class="col-md-7"> <input type="password" class="form-control" name="password" value="${user.password }" /> </div> </div> <div class="form-group "> <input type="submit" class="btn btn-primary" value="Register" /> </div> </form> </div> </c:when> <c:when test="${mode=='ALL_USERS' }"> <div class="container text-center" id="tasksDiv"> <h3>All Users</h3> <hr> <div class="table-responsive"> <table class="table table-striped table-bordered"> <thead> <tr> <th>Id</th> <th>UserName</th> <th>First Name</th> <th>LastName</th> <th>Age</th> <th>Delete</th> <th>Edit</th> </tr> </thead> <tbody> <c:forEach var="user" items="${users }"> <tr> <td>${user.id}</td> <td>${user.username}</td> <td>${user.firstname}</td> <td>${user.lastname}</td> <td>${user.age}</td> <td><a href="/delete-user?id=${user.id }"><span class="glyphicon glyphicon-trash"></span></a></td> <td><a href="/edit-user?id=${user.id }"><span class="glyphicon glyphicon-pencil"></span></a></td> </tr> </c:forEach> </tbody> </table> </div> </div> </c:when> <c:when test="${mode=='MODE_UPDATE' }"> <div class="container text-center"> <h3>Update User</h3> <hr> <form class="form-horizontal" method="POST" action="save-user"> <input type="hidden" name="id" value="${user.id }" /> <div class="form-group"> <label class="control-label col-md-3">Username</label> <div class="col-md-7"> <input type="text" class="form-control" name="username" value="${user.username }" /> </div> </div> <div class="form-group"> <label class="control-label col-md-3">First Name</label> <div class="col-md-7"> <input type="text" class="form-control" name="firstname" value="${user.firstname }" /> </div> </div> <div class="form-group"> <label class="control-label col-md-3">Last Name</label> <div class="col-md-7"> <input type="text" class="form-control" name="lastname" value="${user.lastname }" /> </div> </div> <div class="form-group"> <label class="control-label col-md-3">Age </label> <div class="col-md-3"> <input type="text" class="form-control" name="age" value="${user.age }" /> </div> </div> <div class="form-group"> <label class="control-label col-md-3">Password</label> <div class="col-md-7"> <input type="password" class="form-control" name="password" value="${user.password }" /> </div> </div> <div class="form-group "> <input type="submit" class="btn btn-primary" value="Update" /> </div> </form> </div> </c:when> <c:when test="${mode=='MODE_LOGIN' }"> <div class="container text-center"> <h3>User Login</h3> <hr> <form class="form-horizontal" method="POST" action="/login-user"> <c:if test="${not empty error }"> <div class= "alert alert-danger"> <c:out value="${error }"></c:out> </div> </c:if> <div class="form-group"> <label class="control-label col-md-3">Username</label> <div class="col-md-7"> <input type="text" class="form-control" name="username" value="${user.username }" /> </div> </div> <div class="form-group"> <label class="control-label col-md-3">Password</label> <div class="col-md-7"> <input type="password" class="form-control" name="password" value="${user.password }" /> </div> </div> <div class="form-group "> <input type="submit" class="btn btn-primary" value="Login" /> </div> </form> </div> </c:when> </c:choose> <!-- Optional JavaScript --> <!-- jQuery first, then Popper.js, then Bootstrap JS --> <script src="static/js/jquery-1.11.1.min.js"></script> <script src="static/js/bootstrap.min.js"></script> </body> </html>
Output
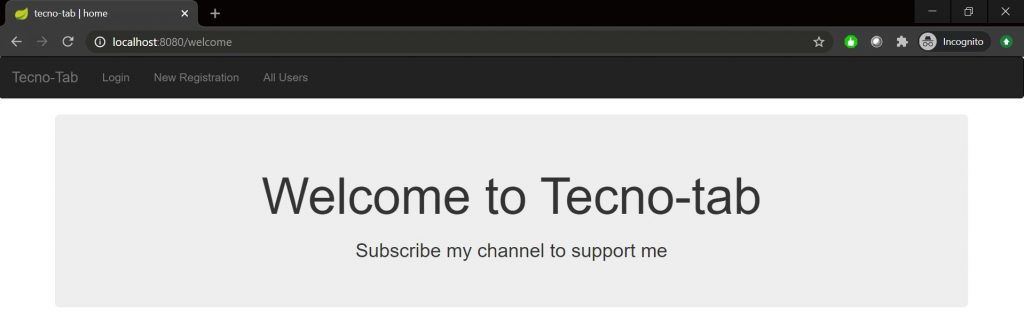
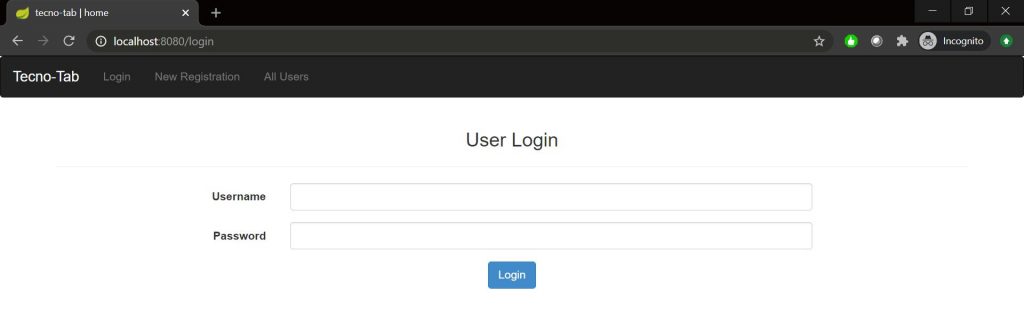
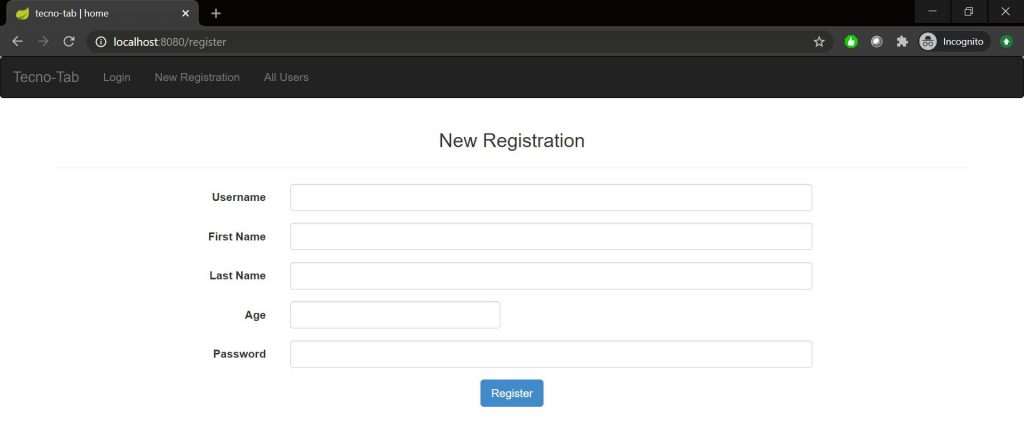
Source Code
You can download the source code Zip by clicking on the Download Zip button or by visiting GitHub.
You can also clone the repository with below link (https), Branch is master.
https://github.com/vikash-kohimam99/MyApplication--SpringBoot-Login-and-Registration-youtube.git
Video Tutorial Link here
Our Other Important Project microservices project with spring boot and spring cloud step by step for beginners.